bone.css
January 17, 2024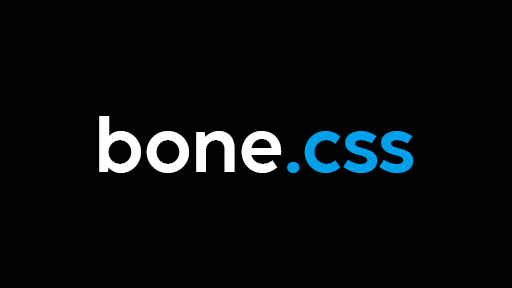
A CSS boilerplate for my personal projects. It has been crafted especially for my needs, but who knows, maybe you find it useful.
Table of Contents
Layout and basics
The layout is based on a 500px width container, inspired from theminimalists.com. The special thing is that I dont use margins or displays for centering the content, but ✨padding✨.
/* With a viewport width of 1000px, */
/* and a max width of 500px, */
/* and a margin of 20px, */
/* obtain max((1000px-500px)/2, 20px) = 250px */
.boned {
--max-width: 500px;
--margin: 20px;
width: 100%;
padding: 0 max(
calc((100% - var(--max-width)) / 2),
var(--margin)
);
}
Using padding for centering
Because of I’m crazy. And because I wanted a way to center an element without depending of a parent container. I wanted to center the element itself. I named it boned
because it’s like a bone, the bone of the viewport.
You can “bone” an element with the class .boned. Body’s header, main and footer are boned by default.
Colors
There are 3 types of colors: white, gray and black. Intensity should be indicated by darker, dark, light or lighter.
Colors will change automatically depending on the user system preference, but if you want to force one theme you need to add the classes .theme-dark
or .theme-light
.
This is an easy rudimentary implementation of switching themes with JavaScript:
const activeThemeDark = () => {
document.body.classList.remove("theme-light");
document.body.classList.add("theme-dark");
};
const activeThemeLight = () => {
document.body.classList.remove("theme-dark");
document.body.classList.add("theme-light");
};
const activeThemePrefersColorScheme = () => {
document.body.classList.remove("theme-dark");
document.body.classList.remove("theme-light");
};
Code
Code snippets are displayed with a monospace font and simple background, like <code>
elements.
const password = "1234";
<pre>
<code>
const <var>password</var> = "1234";
</code>
</pre>
Buttons
Simple. One is active, the other is disabled.
<button>Active</button>
<button disabled>Disabled</button>
It’s easy to add icons and emojis
</button>
<span>📸🤨</span>
Sure?
</button>
<button>
Order
<span>🍕</span>
</button>
Lists
Unordered list
- Zapabunga!
- Petrocombo!
- Treparamas!
- Catalejo!
- Mozirrante!
<ul>
<li>Zapabunga!</li>
<li>Petrocombo!</li>
<li>Treparamas!</li>
<li>Catalejo!</li>
<li>Mozirrante!</li>
</ul>
Tables
A table with columns and rows
Name | Animal | Actions |
---|---|---|
Pelusilla | Cat | ✏️ ❌ |
Bolo | Dog | ✏️ ❌ |
Trocito | Rabbit | ✏️ ❌ |
Cosita | Ferret | ✏️ ❌ |
Juan | Guinea Pig | ✏️ ❌ |
<table class="full">
<thead>
<tr>
<th>Name</th>
<th>Animal</th>
<th style="text-align: end;">Actions</th>
</tr>
</thead>
<tbody>
<tr>
<td>Pelusilla</td>
<td>Cat</td>
<td style="text-align: end;">
<a href="#tables">✏️</a>
<a href="#tables">❌</a>
</td>
</tr>
<tr>
<td>Bolo</td>
<td>Dog</td>
<td style="text-align: end;">
<a href="#tables">✏️</a>
<a href="#tables">❌</a>
</td>
</tr>
<tr>
<td>Trocito</td>
<td>Rabbit</td>
<td style="text-align: end;">
<a href="#tables">✏️</a>
<a href="#tables">❌</a>
</td>
</tr>
<tr>
<td>Cosita</td>
<td>Ferret</td>
<td style="text-align: end;">
<a href="#tables">✏️</a>
<a href="#tables">❌</a>
</td>
</tr>
<tr>
<td>Juan</td>
<td>Guinea Pig</td>
<td style="text-align: end;">
<a href="#tables">✏️</a>
<a href="#tables">❌</a>
</td>
</tr>
</tbody>
</table>
Grids
I usually use
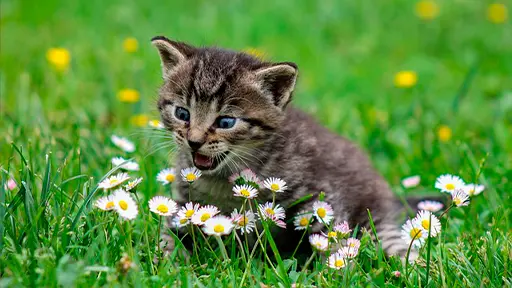
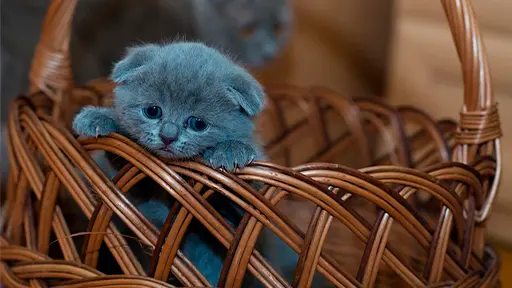
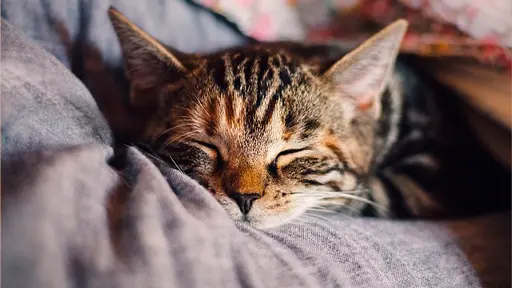
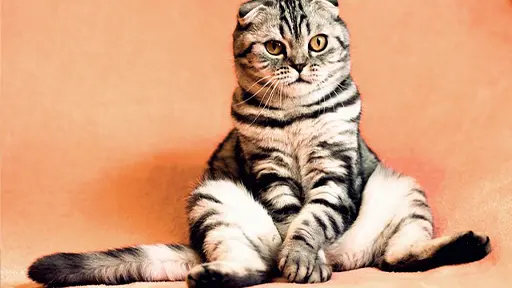
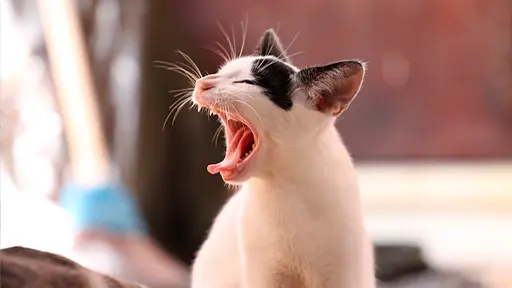
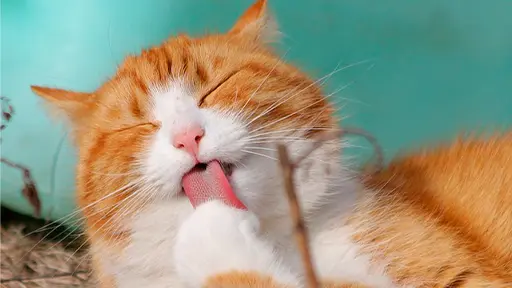
<div class="grid">
<img src="/assets/images/cat-1.webp" alt="cute cat" />
<img src="/assets/images/cat-2.webp" alt="cute cat" />
<img src="/assets/images/cat-3.webp" alt="cute cat" />
<img src="/assets/images/cat-4.webp" alt="cute cat" />
<img src="/assets/images/cat-5.webp" alt="cute cat" />
<img src="/assets/images/cat-6.webp" alt="cute cat" />
</div>
Forms
<form onsubmit="return false;">
<label for="text">
Favourite food
<input
type="text"
name="text"
placeholder="Cookies, bananas, sand..."
/>
</label>
<label for="textarea">
Prove you are not a cat
<textarea
name="textarea"
cols="30"
rows="10"
placeholder="Meow meow meow">
</textarea>
</label>
</form>
Extra classes
Some useful classes.
.boned
For centering the element to the center of the page using padding. Header, main and footer are boned by default.
.full
It applies a width of 100% to the element. Useful for buttons, tables or elements with flex display.
.center
My personal favourite way of centering elements. It applies display: flex, justify-content: center and align-items: center.
.note
Useful for warnings, errors or important messages.
.bordered
Useful for apply a standard border.